Currently, we cannot add tags and categories to our WordPress pages.
Why add tags to pages?
Tags are very useful. They can be applied in a variety of non-standard, but powerful ways to enhance our blogs.
I use tags to filter out file loads and certain types of plugin operations. For example, I only run the wp-syntax plugin functions on pages that actually contain code. Similarly, we can use tags to load in special galleries for particular pages, or restrict a given plugin to a specified group of posts.
This allows us to optimize our WordPress blog and increase page speed.
Tags and categories are also used by plugins to identify related posts (for example the YARPP plugin). Adding tags to pages will presumably help the plugin return better results for both related posts and pages.
How to Add Tags and Categories to WordPress Pages
We can add tags to our WordPress pages by simply using the add_meta_box command as follows –
// Add to the admin_init hook of your theme functions.php file add_meta_box( 'tagsdiv-post_tag', __('Page Tags'), 'post_tags_meta_box', 'page', 'side', 'low');
Argument 1 must be set to tagsdiv-post_tag because this name is used in other WordPress functions to ensure that the metabox operates correctly and the proper tag values get saved with the page.
Argument 3 must be set to post_tags_meta_box because this is the native WordPress function used to render the tag metabox.
Similarly, we can use the same add_meta_box command to add a category metabox to our WordPress page.
add_meta_box( 'categorydiv', __('Categories'), 'post_categories_meta_box', 'page', 'side', 'core');
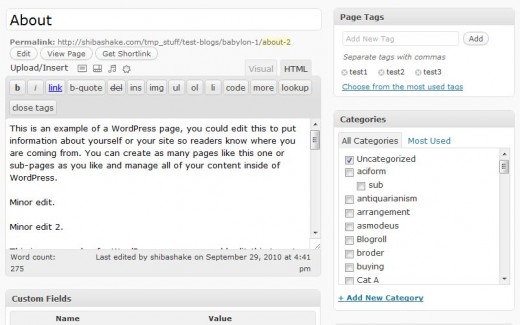
Saving Tags and Categories for WordPress Pages
You will quickly notice that when you update your WordPress page, the category does not get saved.
This is because the WordPress system does a check with the is_object_in_taxonomy function to see if categories can actually be assigned to pages.
To properly save our tag and category data, we want to properly register our WordPress pages to the post_tag and category taxonomies. We can do this with the register_taxonomy_for_object_type function.
// Add to the admin_init hook of your theme functions.php file // Add tag metabox to page add_meta_box( 'tagsdiv-post_tag', __('Page Tags'), 'post_tags_meta_box', 'page', 'side', 'low'); register_taxonomy_for_object_type('post_tag', 'page'); // Add category metabox to page add_meta_box( 'categorydiv', __('Categories'), 'post_categories_meta_box', 'page', 'side', 'core'); register_taxonomy_for_object_type('category', 'page');
Success! Once we do this, our categories get saved with our pages.
Note – As pointed out by XYDAC metaboxes automatically get added as part of the register_taxonomy_for_object_type function. Therefore, we can streamline our code and remove the add_meta_box commands.
// Add to the admin_init hook of your theme functions.php file register_taxonomy_for_object_type('post_tag', 'page'); register_taxonomy_for_object_type('category', 'page');

How to Retrieve Both Posts and Pages for Tag and Category Links
Now that we have set up tags and categories for WordPress pages, we may want our tag and category blog links to also retrieve those pages.
To do this, we can hook into the WordPress request filter.
// Add to the init hook of your theme functions.php file add_filter('request', 'my_expanded_request'); function my_expanded_request($q) { if (isset($q['tag']) || isset($q['category_name'])) $q['post_type'] = array('post', 'page'); return $q; }
We Are Done!
Enjoy your new expanded WordPress pages.
Old post. As of 3.0
add_action('init', 'add_category_metabox');
function add_category_metabox() {
register_taxonomy_for_object_type('category', 'page');
}
is enough
This is probably an obvious one, but can this code be used on a wp.com theme and editing under the customize–>css segment? Trying yet another shot in the dark here and any help would be appreciated.
No, this requires PHP code.
Hey I have created a category in pages section but how to display the pages who add on the particular category
i just cant add category and tag on firefox and chrome browser, if i try add tag and category with opera browser its work. i have reinstall and install firefox and remove all addon firefox but its still not working. I do deactive and active plugin wordpress that is the same, i cant add catergory and tag.
oh ya, this morning i have update wordpress with the last version 4.1