Currently, we cannot add tags and categories to our WordPress pages.
Why add tags to pages?
Tags are very useful. They can be applied in a variety of non-standard, but powerful ways to enhance our blogs.
I use tags to filter out file loads and certain types of plugin operations. For example, I only run the wp-syntax plugin functions on pages that actually contain code. Similarly, we can use tags to load in special galleries for particular pages, or restrict a given plugin to a specified group of posts.
This allows us to optimize our WordPress blog and increase page speed.
Tags and categories are also used by plugins to identify related posts (for example the YARPP plugin). Adding tags to pages will presumably help the plugin return better results for both related posts and pages.
How to Add Tags and Categories to WordPress Pages
We can add tags to our WordPress pages by simply using the add_meta_box command as follows –
// Add to the admin_init hook of your theme functions.php file add_meta_box( 'tagsdiv-post_tag', __('Page Tags'), 'post_tags_meta_box', 'page', 'side', 'low');
Argument 1 must be set to tagsdiv-post_tag because this name is used in other WordPress functions to ensure that the metabox operates correctly and the proper tag values get saved with the page.
Argument 3 must be set to post_tags_meta_box because this is the native WordPress function used to render the tag metabox.
Similarly, we can use the same add_meta_box command to add a category metabox to our WordPress page.
add_meta_box( 'categorydiv', __('Categories'), 'post_categories_meta_box', 'page', 'side', 'core');
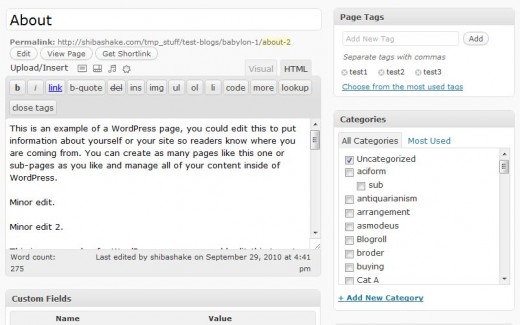
Saving Tags and Categories for WordPress Pages
You will quickly notice that when you update your WordPress page, the category does not get saved.
This is because the WordPress system does a check with the is_object_in_taxonomy function to see if categories can actually be assigned to pages.
To properly save our tag and category data, we want to properly register our WordPress pages to the post_tag and category taxonomies. We can do this with the register_taxonomy_for_object_type function.
// Add to the admin_init hook of your theme functions.php file // Add tag metabox to page add_meta_box( 'tagsdiv-post_tag', __('Page Tags'), 'post_tags_meta_box', 'page', 'side', 'low'); register_taxonomy_for_object_type('post_tag', 'page'); // Add category metabox to page add_meta_box( 'categorydiv', __('Categories'), 'post_categories_meta_box', 'page', 'side', 'core'); register_taxonomy_for_object_type('category', 'page');
Success! Once we do this, our categories get saved with our pages.
Note – As pointed out by XYDAC metaboxes automatically get added as part of the register_taxonomy_for_object_type function. Therefore, we can streamline our code and remove the add_meta_box commands.
// Add to the admin_init hook of your theme functions.php file register_taxonomy_for_object_type('post_tag', 'page'); register_taxonomy_for_object_type('category', 'page');

How to Retrieve Both Posts and Pages for Tag and Category Links
Now that we have set up tags and categories for WordPress pages, we may want our tag and category blog links to also retrieve those pages.
To do this, we can hook into the WordPress request filter.
// Add to the init hook of your theme functions.php file add_filter('request', 'my_expanded_request'); function my_expanded_request($q) { if (isset($q['tag']) || isset($q['category_name'])) $q['post_type'] = array('post', 'page'); return $q; }
We Are Done!
Enjoy your new expanded WordPress pages.
Works like a charm! Thank you.
how do you retrieve attached categories of front page?
The Code
Hi,
I add your suggested code in my source code to add Category on my pages.
Now, I’d like to create a sidebar that show pages of a similar Category.
Can you help me, please?
Thank you.
Simone
Great! Thanks!
Thanks so much, this fixed my error (Call to undefined function add_meta_box()).
Here’s the completed code that works and saves the cats and tags.
how do i show pages from one category? query posts doesn’t seem to go looking for pages. ??
One possibility is to hook into the ‘request’ filter.
http://shibashake.com/wordpress-theme/mastering-the-wordpress-loop
I think I have the same problem than Jaap: It works fine, I can add tags and categories to the pages, but when I call the categories whithin the loop (with the_category()) to show them on the page they don’t appear, but tags do. Somebody knows how can I show them? Thanks
This is awesome! Thank you. Will our permalink structure settings apply to pages? Like if we set /%category%/%post%/, will that also result in /%category%/%page%/? Can we do this with Yoast’s SEO Plugin?
No. You will have to manually set this if you want to change page permalinks.
Don’t know. I have never used this plugin.
This seems to be play happy with latest WP:
And how i display the tags on my theme?
Hi,
Thanks for this code, i was looking for this kind of plugin where i can add categories with my pages. Although it is not exactly what i need but a bit helpful. I have a question :
Is there any way using your code or some extra bit of code, we can retrieve only specific category and it’s subcategories and show on pages the same way you are showing on “add page” section ?
Please help.
Thanks
Vivek
Yes. I would probably just copy the existing meta-box code for categories, and then alter that to only show the categories you want. Then use add_meta_box to add it to the page.
I am trying to implement this. I am using WordPress 3.2.1. When I add the code above to the functions.php, it returns an error Call to undefined function add_meta_box(). I am using the TwentyTen themes function.php. Is this just because I am putting it in the wrong place or what?
Thanks
It could be that you included the function call before the proper file was loaded. Check out the WordPress Codex for a detailed example of where to add the add_meta_box function call.
http://codex.wordpress.org/Function_Reference/add_meta_box
Hi !
I would like , how to add Category meta box and my admin page ?
I have developped my own plugin and i need to use WP category and i want to display div id= “categorydiv” in my admin_plugin page?
Its possible ?
http://shibashake.com/wordpress-theme/standard-wordpress-metabox