As of WordPress 3.3, the Edit Comments Screen allows us to change the name, email, url, status, and text of a blog comment. If we are using the Akismet spam filter, then there will also be a Comment History metabox.
Here, we describe how we can expand the Edit Comments Screen by adding a new metabox.
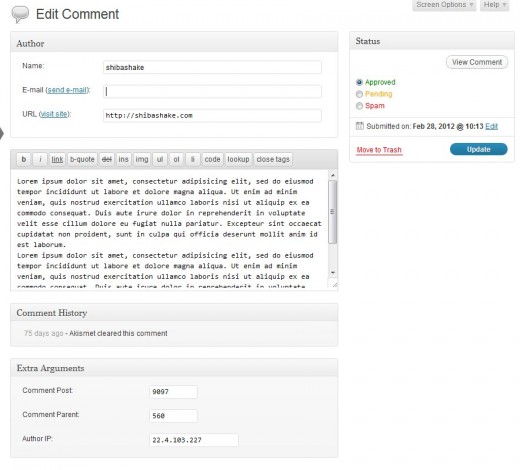
For example, in the screenshot above, we added an Extra Arguments metabox to the Edit Comments screen. It allows us to change the –
- Comment Post – By changing the post ID that is associated with the comment, we can move the comment from one post or page to any other post of our choice. In fact, we could move it from any post type (e.g. post, page, custom post type) to another.
- Comment Parent – Changing this value allows us to thread a comment, or remove the comment from a thread (by setting its parent to 0).
- Author IP – Change the comment IP.
1. Create and Add Metabox
We start by creating our metabox input elements and then adding it using the WordPress add_meta_box function.
// Add to the admin_init function add_meta_box('shiba_comment_xtra_box', __('Extra Arguments'), 'my_metabox', 'comment', 'normal');
Note – As of WordPress 3.3 new comment metaboxes can only be added to the normal context. I.e., argument 5 must be set to ‘normal’ for our metabox to be rendered.
In addition to adding our metabox, we must also create it in our my_metabox function (argument 3). The metabox elements below were created by adapting the existing Author metabox, at the top of the Edit Comments screen. This code can be found in the wp-admin/edit-form-comment.php file.
function my_metabox($comment) { ?> <table class="form-table editcomment comment_xtra"> <tbody> <tr valign="top"> <td class="first"><?php _e( 'Comment Post:' ); ?></td> <td><input type="text" id="shiba_comment_post_ID" name="shiba_comment_post_ID" size="10" class="code" value="<?php echo esc_attr($comment->comment_post_ID); ?>" tabindex="1" /></td> </tr> <tr valign="top"> <td class="first"><?php _e( 'Comment Parent:' ); ?></td> <td><input type="text" id="shiba_comment_parent" name="shiba_comment_parent" size="10" class="code" value="<?php echo esc_attr($comment->comment_parent); ?>" tabindex="1" /></td> </tr> <tr valign="top"> <td class="first"><?php _e( 'Author IP:' ); ?></td> <td><input type="text" id="shiba_comment_author_IP" name="shiba_comment_author_IP" size="20" class="code" value="<?php echo esc_attr($comment->comment_author_IP); ?>" tabindex="1" /></td> </tr> </tbody> </table> <?php }
The code above creates three new text inputs for our metabox, populates it with the relevant values, and adds it to the Edit Comments screen.
Now, all we need to do is save our added input values.
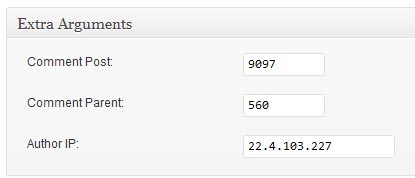
2. Save Metabox Values
To save our extra comment values, we want to hook into the comment_save_pre filter. When a comment gets created or updated, the edit_comment function is called. This function performs the proper security checks and calls wp_update_comment which in turn applies the comment_save_pre filter.
add_meta_box('shiba_comment_xtra_box', __('Extra Arguments'), array(&$this,'comment_xtra_metabox'), 'comment', 'normal'); add_filter('comment_save_pre', 'save_mycomment_data' );
Once we hook into wp_update_comment function, we can save our additional comment values by doing the following –
function save_mycomment_data($comment_content) { global $wpdb; $comment_post_ID = absint($_POST['shiba_comment_post_ID']); $comment_parent = absint($_POST['shiba_comment_parent']); $comment_author_IP = esc_attr($_POST['shiba_comment_author_IP']); $comment_ID = absint($_POST['comment_ID']); // comment parent cannot be self if ($comment_parent == $comment_ID) return $comment_content; // don't update // check that post ID exists $post = get_post($comment_post_ID); if (!$post) return $comment_content; // don't update // From wp_update_comment $data = compact('comment_post_ID', 'comment_parent', 'comment_author_IP'); $rval = $wpdb->update( $wpdb->comments, $data, compact( 'comment_ID' ) ); wp_update_comment_count($comment_post_ID); return $comment_content; }
Lines 4-7 – Extract and clean the relevant comment values from our submitted form.
Lines 9-15 – Check that comment parent id and comment post id values are valid. If not, then do not update comment.
Lines 17-19 – Update the comment in our WordPress database.
Line 21 – Update the comment count for the post we are moving our comment to.
And that is all we need to do to expand the Edit Comments screen.
How can I remove the core author metabox from Edit Comments Screen?
Hi,
Thanks for the tip, useful. Interesting to know that ‘comment’ is not one of the options for “post_type” when calling “add_meta_box”, 4th argument.
Also, you forgot to mention that you need to hook the add_meta_boxes before you can call the “add_meta_box” function you created as in:
add_action( ‘add_meta_boxes’, ‘add_meta_boxes’ );
function add_meta_boxes()
{
// Add to the admin_init function
add_meta_box(‘shiba_comment_xtra_box’, __(‘Extra Arguments’), ‘my_metabox’, ‘comment’, ‘normal’);
}
Hello,
Can you give me a hint how can I remove the ‘namediv’ metabox from this editcomment screen?
Hello,
Thanks for this great tutorial. I want to give comment author to edit his own comment. So need to disable the name, email and url field from the Author metabox also disable to edit the comment date and time to edit. But not with css want to do it with filter or hook.
Comment author can just update the content.
I tried to remove the metabox with remove_meta_box( ‘namediv’ , ‘comment’ , ‘normal’ );
but did not work.
Can tell me how can I do it?
Thanks for this tutorial, Shiba. I just have a question for the sake of understanding and learning more.
Why does the ‘save_mycomment_data’ function takes in $comment_content as a parameter? Can it take $comment or $comment_id as a parameter?
For most tutorials on adding meta boxes on pages or posts, the save data function always takes in $post_id as a parameter. Why is it different on saving comment data? Thanks in advance for your answer!
Hello Ren,
The arguments passed in depends on which WordPress hook we use. In the example above, I used the ‘comment_save_pre’ hook, which passes back the comment content argument. This is set in WP native code. Here is more on WordPress hooks.
Hi,
Thanks for posting on extend metabox in commend edit screen.
It really works. but how to extend it in comment metabox when you at post edit screen?
Thank you.
I do my comment edits on the comment admin screens so this is not something I have looked at.
Update – I checked it out and all you need to do is expand the screen check condition to include the post screen. For example-