In Part-1 of this series, we described how to add a simple media manager popup for selecting an image. In addition to image selection, there are many other useful media manager functions, for example, the gallery editing interface.
In this article, we explore how to access and customize some of these additional media manager functions. In particular, we want to add the Edit Gallery function, from the media manager interface, into our Media Library plugin. We describe how to do that here.
Custom-Header.js File
We can get a lot of insight into how to create a media manager frame by looking at the custom-header.js file. Of particular interest, is the line which contains –
1 | frame = wp.media.frames.customHeader = wp.media({ ... }); |
This is where the media manager frame gets created. We can do some simple customization to the frame by setting various attributes in the wp.media function call.
For example, in the custom-header.js file, we customize the title, library (type), and button of the media manager frame.
1. Create Our Own Media Manager Frame
To more fully customize our media manager interface, we start by creating our own frame (similar to what was done in the custom-header.js file.
1 | wp.media.shibaMlibEditGallery = { |
2 | |
3 | frame: function () { |
4 | if ( this ._frame ) |
5 | return this ._frame; |
6 |
7 | this ._frame = wp.media({ |
8 | id: 'my-frame' , |
9 | frame: 'post' , |
10 | state: 'gallery-edit' , |
11 | title: wp.media.view.l10n.editGalleryTitle, |
12 | editing: true , |
13 | multiple: true , |
14 | }); |
15 | return this ._frame; |
16 | }, |
17 |
18 | init: function () { |
19 | $( '#upload-and-attach-link' ).click( function ( event ) { |
20 | event.preventDefault(); |
21 |
22 | wp.media.shibaMlibEditGallery.frame().open(); |
23 |
24 | }); |
25 | } |
26 | }; |
27 |
28 | $(document).ready( function (){ |
29 | $( wp.media.shibaMlibEditGallery.init ); |
30 | }); |
Line 9 – We set the frame type. There are two types of pre-built frames in WordPress 3.5 – post and select. Select is the default frame type and it is the interface that is created in the custom-header.js file.
The post frame is what appears when we click on the Add Media button while editing posts or pages.
For more detail on these two frame types, we can look at MediaFrame.Post and MediaFrame.Select in the wp-includes/js/media-views.js file.
Line 10 – We set the state to ‘gallery-edit’ so that when we open the media manager interface, we are on the edit gallery screen.
Line 11 – Use the default edit gallery screen title.
Line 12 – Allow gallery to be edited.
Line 13 – Allow multiple images to be selected.
Line 19 – Bind the frame to an interface button. In this case, we open the media manager interface when our HTML button with id=”upload-and-attach-link” gets clicked.
Line 20 – Do not allow the click to do anything else.
Line 22 – Open our media manager frame.
When we click on our #upload-and-attach-link button, we get the media-manager frame below.

2. Get Initial Gallery Images
Note that the Edit Gallery frame above always appears as blank. To fill the frame with an initial set of images, we must set the selection attribute while creating our frame.
1 | var selection = this .select(); |
2 | this ._frame = wp.media({ |
3 | id: 'my-frame' , |
4 | frame: 'post' , |
5 | state: 'gallery-edit' , |
6 | title: wp.media.view.l10n.editGalleryTitle, |
7 | editing: true , |
8 | multiple: true , |
9 | selection: selection |
10 | }); |
We must also write the select function to create a selection of images. One common way to populate our gallery is through its gallery shortcode. There is already code that does this in the media-editor.js file. Below, we adapt the native code for our own purposes.
1 | // Gets initial gallery-edit images. Function modified from wp.media.gallery.edit |
2 | // in wp-includes/js/media-editor.js.source.html |
3 | select: function () { |
4 | var shortcode = wp.shortcode.next( 'gallery' , wp.media.view.settings.shibaMlib.shortcode ), |
5 | defaultPostId = wp.media.gallery.defaults.id, |
6 | attachments, selection; |
7 |
8 | // Bail if we didn't match the shortcode or all of the content. |
9 | if ( ! shortcode ) |
10 | return ; |
11 |
12 | // Ignore the rest of the match object. |
13 | shortcode = shortcode.shortcode; |
14 |
15 | if ( _.isUndefined( shortcode.get( 'id' ) ) && ! _.isUndefined( defaultPostId ) ) |
16 | shortcode.set( 'id' , defaultPostId ); |
17 |
18 | attachments = wp.media.gallery.attachments( shortcode ); |
19 | selection = new wp.media.model.Selection( attachments.models, { |
20 | props: attachments.props.toJSON(), |
21 | multiple: true |
22 | }); |
23 | |
24 | selection.gallery = attachments.gallery; |
25 |
26 | // Fetch the query's attachments, and then break ties from the |
27 | // query to allow for sorting. |
28 | selection.more().done( function () { |
29 | // Break ties with the query. |
30 | selection.props.set({ query: false }); |
31 | selection.unmirror(); |
32 | selection.props.unset( 'orderby' ); |
33 | }); |
34 |
35 | return selection; |
36 | }, |
This select function returns a wp.media.model.Selection object that contains all the images for the shortcode string that is defined in wp.media.view.settings.shibaMlib.shortcode.
We previously set this variable (in php) by hooking into the media_view_settings filter. This filter is used to pass attributes from our server (php) into the media manager interface (javascript).
1 | add_filter( 'media_view_settings' , array ( $this , 'media_view_settings' ), 10, 2 ); |
2 |
3 | function media_view_settings( $settings , $post ) { |
4 | if (! is_object ( $post )) return $settings ; |
5 |
6 | // Create our own shortcode string here |
7 | $shortcode = ... |
8 |
9 | $settings [ 'shibaMlib' ] = array ( 'shortcode' => $shortcode ); |
10 | return $settings ; |
11 | } |
Once we define a selection, our Edit Gallery menu will start with our defined set of images (shown below).
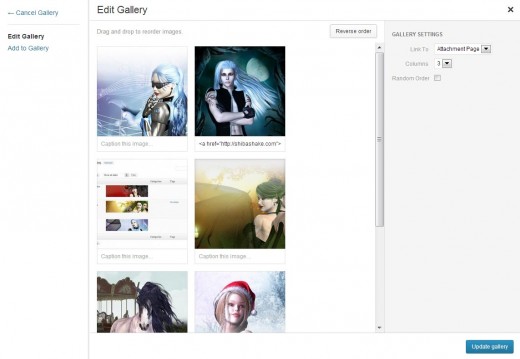
3. Specify Our Frame Update Function
Now, all we need to do, is to write our own update function for when we click on the Update Gallery button.
1 | this ._frame.on( 'update' , |
2 | function () { |
3 | var controller = wp.media.shibaMlibEditGallery._frame.states.get( 'gallery-edit' ); |
4 | var library = controller.get( 'library' ); |
5 | // Need to get all the attachment ids for gallery |
6 | var ids = library.pluck( 'id' ); |
7 |
8 | // send ids to server |
9 | wp.media.post( 'shiba-mlib-gallery-update' , { |
10 | nonce: wp.media.view.settings.post.nonce, |
11 | html: wp.media.shibaMlibEditGallery.link, |
12 | post_id: wp.media.view.settings.post.id, |
13 | ids: ids |
14 | }).done( function () { |
15 | window.location = wp.media.shibaMlibEditGallery.link; |
16 | }); |
17 |
18 | }); |
Lines 3-6 – Extract the list of image-ids associated with our current Edit Gallery menu.
Lines 8-14 – Send the list of image-ids to the server to be processed. ‘shiba-mlib-gallery-update’ is our own AJAX function id. We can link our own server function to it, by doing the following.
1 | add_action( 'wp_ajax_shiba-mlib-gallery-update' , |
2 | array ( $this , 'wp_ajax_shiba_mlib_gallery_update' )); |
Lines 15 – Refresh our gallery object screen once we finish our AJAX function call.
We can add our update bind-event to our earlier frame creation function. For example, right before
1 | return this ._frame; |
We Are Done!
We can use the process above to access other post frame menus, customize update operations, and further customize the media manager interface according to our needs.
To every people who are stucked, as i was, since two days. (i use WordPress 4.5.3)
Replace : if (!is_object($post)) return $settings;
By: if ( isset( get_current_screen()->post_type ) && (get_current_screen()->post_type == ‘YOUR POST TYPE’ ) ) {
$post = get_post( $_GET[‘post’] );
}
Explanation: If you use a custom post type like i was ‘$post’ will always be a non-object and you’re not gonna be able to pass the if.
—
For those who don’t get how to have a shortcode string, that’s simple. Juste use a basic shortcode like : [gallery ids="729,732,731,720"]. The numbers are ids of attachments you want to show.
Here is my personal code. It’s not gonna resolve all issues but it’s easily adaptable:
$imageIdsArray = json_decode(get_post_meta($post->ID, “_gallery_medias_ids”, true));
// i previously saved my images using json_encode (to transform my array of // ids into a string like ‘[729,732]’ in database
if( !empty( $imageIdsArray ) ) {
$imageIds = implode($imageIdsArray, ‘ , ‘);
$shortcode = ‘[gallery ids="'. $imageIds .'"]‘;
$settings[‘myGallery’] = array(‘shortcode’ => $shortcode);
}
return $settings;
—
Finally in your .js file put:
var shortcode = wp.shortcode.next( ‘gallery’, wp.media.view.settings.myGallery.shortcode )
And you’ll be fine 🙂
—
Thanks to the author ! You made the most comprehensible documentation about wp.media manipulation and i’m writing this comment in 2016 (!!)
Hi realy a good tutorian thank you 🙂
A question, I wuold like to keep the “select a file” button open even when there are file already loaded in the mediamanager. Is there a way to do this?
Thank you
Hi, I got lost at this part:
// Create our own shortcode string here
$shortcode = ...
How do I generate the shortcode?
Nice tutorial ShibaShake.
I’m stuck with this:
wp.media.post( ‘shiba-mlib-gallery-update’, {
nonce: wp.media.view.settings.post.nonce,
html: wp.media.shibaMlibEditGallery.link,
post_id: wp.media.view.settings.post.id,
ids: ids
}).done( function() {
window.location = wp.media.shibaMlibEditGallery.link;
});
and this:
add_action( ‘wp_ajax_shiba-mlib-gallery-update’,
array($this,’wp_ajax_shiba_mlib_gallery_update’));
wp.media.shibaMlibEditGallery.link returns undefined.
Do you mind giving an example setting of wp.media.shibaMlibEditGallery.link and implementation of wp_ajax_shiba_mlib_gallery_update.
I really appreciate it so much. Thanks again 🙂
Nice tutorial, thanks. Helped me fix an issue in my plugin. 😉
Hi, nice article, im running the media uploader window from a colorbox modal window, which i set to 90%width, and inside i have button which trigger the media uploader.
but when i trigger it, it everytime opens smaller than the parent colorbox modal.
where can i set the width and height for the window with media uploader?
thanks for reply.
Hi there,
I do not properly understand the 2nd part (2. Get Initial Gallery Images) at all. I am not using your plugin/theme, but want to use the code on the front end. I mastered step 1 ok – so I get the media modal, but there are no proper explanations for step 2. I would like to only show the uploaded images to the post that is just being viewed. Is there a good way to do this? Apart from that the explanations are very detailed and very helpful!
Thanks,
Sascha
i get error when adding this code
wp.media.view.settings.shibaMlib.shortcode
please help
Hi
Thank you for making this tutorial!
I get an error when I try to add the shortcode:
TypeError: ‘undefined’ is not an object (evaluating ‘wp.media.view.settings.shibaMlib.shortcode’)
select (media-uploader.js, line 23)
frame (media-uploader.js, line 9)
(anonymous function) (media-uploader.js, line 61)
dispatch (load-scripts.php, line 4)
handle (load-scripts.php, line 4)
Can you please help me?
Thanks
Michael