The WordPress Quick Edit menu allows us to quickly modify certain object attributes without having to go into the full object Edit Screen. The Quick Edit menu uses javascript to display the edit interface, thereby saving us time and additional calls to our WordPress server.

The first step towards expanding our WordPress Quick Edit Menu is to add a custom column to our target Edit Screen. In this example tutorial, we will expand the Quick Edit menu for Post objects. Therefore, we start by adding a custom column to our Posts screen.
1. Add a Custom Column
We add a custom column called Widget Set which shows some custom meta data associated with our Post objects.
// Add to our admin_init function add_filter('manage_post_posts_columns', 'shiba_add_post_columns'); function shiba_add_post_columns($columns) { $columns['widget_set'] = 'Widget Set'; return $columns; }
The code above adds a new but empty column to our Posts screen.

To fill our new column with the relevant meta-data values, we use the manage_posts_custom_column action hook.
// Add to our admin_init function add_action('manage_posts_custom_column', 'shiba_render_post_columns', 10, 2); function shiba_render_post_columns($column_name, $id) { switch ($column_name) { case 'widget_set': // show widget set $widget_id = get_post_meta( $id, 'post_widget', TRUE); $widget_set = NULL; if ($widget_id) $widget_set = get_post($widget_id); if (is_object($widget_set)) echo $widget_set->post_title; else echo 'None'; break; } }
Line 2 – Add the manage_posts_custom_column action hook to our admin_init function.
Lines 5 to 6 – Check for our new custom column.
Lines 8 to 13 – Render our custom Post object meta-data values.
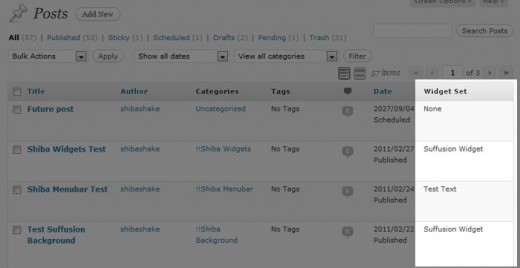
Now, our new column is filled with the relevant meta-data values.
2. Expand the WordPress Quick Edit Menu
After adding our custom column, we are ready to expand our Post Quick Edit menu using the quick_edit_custom_box action hook.
Note – The quick_edit_custom_box action hook will not fire unless there are custom columns present. That is why we started by adding a custom column.
// Add to our admin_init function add_action('quick_edit_custom_box', 'shiba_add_quick_edit', 10, 2); function shiba_add_quick_edit($column_name, $post_type) { if ($column_name != 'widget_set') return; ?> <fieldset class="inline-edit-col-left"> <div class="inline-edit-col"> <span class="title">Widget Set</span> <input type="hidden" name="shiba_widget_set_noncename" id="shiba_widget_set_noncename" value="" /> <?php // Get all widget sets $widget_sets = get_posts( array( 'post_type' => 'widget_set', 'numberposts' => -1, 'post_status' => 'publish') ); ?> <select name='post_widget_set' id='post_widget_set'> <option class='widget-option' value='0'>None</option> <?php foreach ($widget_sets as $widget_set) { echo "<option class='widget-option' value='{$widget_set->ID}'>{$widget_set->post_title}</option>\n"; } ?> </select> </div> </fieldset> <?php }
Line 5 – Only render our Quick Edit extension on the relevant screen.
Lines 7 to 25 – Render our custom drop-down menu for selecting widget sets.
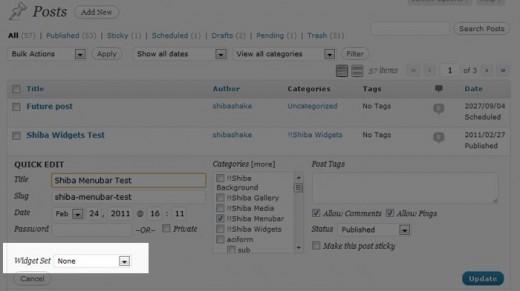
3. Save Custom Quick Edit Data
We save our new quick edit menu data in the same way that we save new custom meta-box data – through the save_post action hook. The save_post function below was adapted from the example found on WordPress.org.
// Add to our admin_init function add_action('save_post', 'shiba_save_quick_edit_data'); function shiba_save_quick_edit_data($post_id) { // verify if this is an auto save routine. If it is our form has not been submitted, so we dont want // to do anything if ( defined('DOING_AUTOSAVE') && DOING_AUTOSAVE ) return $post_id; // Check permissions if ( 'page' == $_POST['post_type'] ) { if ( !current_user_can( 'edit_page', $post_id ) ) return $post_id; } else { if ( !current_user_can( 'edit_post', $post_id ) ) return $post_id; } // OK, we're authenticated: we need to find and save the data $post = get_post($post_id); if (isset($_POST['post_widget_set']) && ($post->post_type != 'revision')) { $widget_set_id = esc_attr($_POST['post_widget_set']); if ($widget_set_id) update_post_meta( $post_id, 'post_widget', $widget_set_id); else delete_post_meta( $post_id, 'post_widget'); } return $widget_set_id; }
4. Update the Quick Edit Menu with Javascript
Now comes the really fun part – notice that while our new custom input shows up in the Quick Edit menu, it is not properly updated to show which ‘widget_set‘ the current Post object is set to. I.e., our widget set meta-data is not getting through to our custom Quick Edit menu.
The set_inline_widget_set javascript function below updates our Quick Edit menu with the relevant meta-data.
// Add to our admin_init function add_action('admin_footer', 'shiba_quick_edit_javascript'); function shiba_quick_edit_javascript() { global $current_screen; if (($current_screen->id != 'edit-post') || ($current_screen->post_type != 'post')) return; ?> <script type="text/javascript"> <!-- function set_inline_widget_set(widgetSet, nonce) { // revert Quick Edit menu so that it refreshes properly inlineEditPost.revert(); var widgetInput = document.getElementById('post_widget_set'); var nonceInput = document.getElementById('shiba_widget_set_noncename'); nonceInput.value = nonce; // check option manually for (i = 0; i < widgetInput.options.length; i++) { if (widgetInput.options[i].value == widgetSet) { widgetInput.options[i].setAttribute("selected", "selected"); } else { widgetInput.options[i].removeAttribute("selected"); } } } //--> </script> <?php }
Lines 5 to 6 – Only add the javascript quick edit function to the Posts screen.
Line 13 – Make sure that Quick Edit menu is closed. The revert function (defined in inline-edit-post.js) ensures that our Quick Edit custom inputs are properly updated when we switch Post objects.
Lines 15 to 16 – Set nonce value for our custom inputs. If we want, we can expand our save_post function to do a nonce check.
Lines 18 to 22 – Set the proper widget set option on our custom Quick Edit drop-down menu.
5. Link Javascript to the Quick Edit Link
Finally, we want to link our set_inline_widget_set javascript function to the Quick Edit link so that it will update all our custom input values when a Quick Edit link is clicked.
We do this by hooking into the post_row_actions filter. Post object link actions are originally defined in the WP_Posts_List_Table class.
// Add to our admin_init function add_filter('post_row_actions', 'shiba_expand_quick_edit_link', 10, 2); function shiba_expand_quick_edit_link($actions, $post) { global $current_screen; if (($current_screen->id != 'edit-post') || ($current_screen->post_type != 'post')) return $actions; $nonce = wp_create_nonce( 'shiba_widget_set'.$post->ID); $widget_id = get_post_meta( $post->ID, 'post_widget', TRUE); $actions['inline hide-if-no-js'] = '<a href="#" class="editinline" title="'; $actions['inline hide-if-no-js'] .= esc_attr( __( 'Edit this item inline' ) ) . '" '; $actions['inline hide-if-no-js'] .= " onclick=\"set_inline_widget_set('{$widget_id}', '{$nonce}')\">"; $actions['inline hide-if-no-js'] .= __( 'Quick Edit' ); $actions['inline hide-if-no-js'] .= '</a>'; return $actions; }
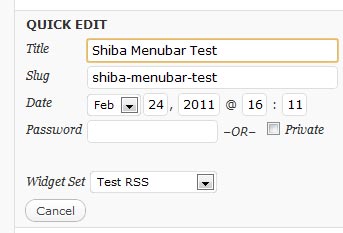
Lines 5 to 6 – Only expand Quick Edit links for the Posts screen.
Lines 8 to 9 – Get nonce and other custom input values associated with the current post.
Line 12 – Add the onclick event to our Quick Edit link and associate it to our set_inline_widget_set javascript function.
Related Quick Edit Menu Source Files
- Javascript Quick Edit file for post objects.
- inline_edit – Renders the HTML Quick Edit menu form.
- get_inline_data – Generates data that is later used by the javascript inline editor for posts and pages.
- Quick Edit link action.
hi, tanks for this post …
but i have a question … Is it possible that change custome feild data in in line edit in wordpress panel and how add this form …!!!?
Brilliant!
Followed your tut line by line and adapted to my needs (adding a custom column with each linked Mailpress draft’s title). Everything worked like a charm! Thanks a ton, mainly because I feel I’ve learned a lot while reading your code, editing it and debugging my mistakes. That’s unvaluable.
Cheers,
rash*
Sorry, I “feel” it will work. But it does´nt. It is the POST_TYPE. But I like to have a sidebar (widgets) selection. What is to do?
Thanks!
Mark from Germany.
This is a very long time away from your original comment, but did you ever work it out? I am stuck on the exact same thing four years on!
Hi.
Question:
I’m testing this article in my WP installation.
For testing purposes, I’ve modified the code to do the following:
add_action(‘save_post’, ‘shiba_save_quick_edit_data’);
function shiba_save_quick_edit_data($post_id) {
(…)
// OK, we’re authenticated: we need to find and save the data
if (isset($_POST[‘post_widget_set’]) && ($post->post_type != ‘revision’)) {
echo ‘Show this’;
}
return $widget_set_id;
}
The problem is “Show this text” is printing two times.
This is a problem because I’m trying to insert data in my database and I’m getting duplicates.
Is there a way to have only one “Show this” text?
Thankyou so much!
Hi, Thanks for this post shiba, it help me so much but,
I need insert checkboxes, in the dropdown only I choose a option, but in the checkboxes, I can choose multiple options . How can I do the change?
Examples for several input types would be amazing. I tried to get this with just a simple text field and it took quite a bit. Also I have no idea how to get the JS part working, its very deep. Thanks for this TUT!
Hey, thanks for the walkthrough. Really helpful, fantastic research.
One thing… any idea how it’s possible to add the quickedit box to a different column? At the moment it just dumps it at the end of the quickedit html and sits below everything else.
Josh
The entire quick edit menu is rendered in the inline_edit function.
I did a quick scan through the function and didn’t see any obvious way to flexibly change the quickedit menu structure through hooks.
Two possible options-
1. Add in empty fieldset cells to skip columns.
2. Use javascript.
Hey All,
If you want to prepopulate your field with jQuery it’s much simpler. Just inclue this script after your fieldset.
;(function($) {
jQuery(‘tr.inline-edit-row .keywords’).val(jQuery(‘tr.inline-edit-row’).prev(‘tr.type-equipment’).find(‘td.keywords’).text());
})(jQuery);
I dont understand this script, can you explain to me? please
thank u first
then my ask:
my onclick=\”set_inline_slider1()\”
remove after i click on update button.
what’s the problem.
in oder word
my quck edit link just have first value of my custom field and not refresh value after update
thanks a lot
Thanks so much – I’ve got further with this than any other tutorial and think I will get it working, but I really need it for pages and custom post types more than standard posts. Could you tell me which fields I should be looking to update for this to work. Also, would I need to add this code to my functions file 3 times if I wanted it to work for 3 types of post? Sorry for complete idiocy, but this is such a great function I really want to understand it!